What’s with All the Weird Slashes and Semicolons and Stuff?
So, as you may have figured out by now, code is weird to start reading and writing if you’ve never seen it before. There are a whole bunch of symbols all over the place that probably don’t make sense to you right now. Let’s take a little time to figure out some of the ones you’re going to be seeing over and over again. They’re all there for a reason and when you’re missing even just one of them the computer or robot won’t understand what you’ve written! Here’s what we’ll be covering in this lesson:Comments: They Help! (No Really, They Seriously Do)
Ok. Take a deep breathe, if this is your first time to write code you first need to realize that it’s like learning a new language (it actually is a new language). It’s going to be a while before you can easily read and write in this language, even longer before you can write novels or poems. But it’s definitely worth it! With your new found language you will be able to control robots, video games, internet servers and communicate all over the world.
1 2 3 4 5 |
// this is a comment /* this is also a comment */ |
1 2 3 4 5 6 7 |
void loop() { sparki.moveForward(); //sparki.moveRight(); sparki.moveLeft(); sparki.moveBackward(); } |
Semicolons: (They’re not just a wink)
You’ve probably noticed the semicolons everywhere in the code you’re learning how to write. If you haven’t noticed them, take a look at any of the examples of code supplied on this website- they’re everywhere! So what exactly do they mean? Semicolons are basically a way for the robot or computer to understand that it has come to the end (the termination) of a simple set of instructions and that it should have enough information to execute a complete command. For this reason semicolons are referred to as a statement terminator. They tend to come at the end of almost every line of code except for loop related lines of code. You’ll see them at the end of all the commands that declare (create) or assign (change) variables. You’ll also see them at the end of lines that use input or output commands to work with things like sensors and motors.
1 2 3 4 5 6 7 |
void loop() // code inside these brackets runs over and over forever { sparki.moveForward(); //look at that semicolon! sparki.moveRight(); //woah, another one. sparki.moveLeft(); //what's that at the end of this line? Gasp! A semicolon. sparki.moveBackward(); //I think you get the point. } //the robot goes back to the top of the loop once it reaches here |
Parentheses: (with Ketchup)
Parenthesis are usually used in one of two ways- either to ask questions (these are called conditional) or to pass information to chunks of code. To ask a question with parentheses a programmer will type a variable or equation inside of a set of parentheses, if the variable or equation is equal to true then the code that comes after the question in the parentheses is executed. If statements are a perfect example of this usage of parentheses. When passing information using parenthesis the parentheses usually come after a function command. You can even pass multiple pieces of information inside a single set of parentheses! The function then takes this information and uses it somehow in the chunk of code the function executes. For example, a sparki.moveForward(10); command will pass the number ten to the code inside the moveForward function making Sparki move forward ten centimeters. If the function was passed a value of two, instead of ten, then Sparki would only move forward two centimeters. Anytime there is a left hand parenthesis ‘(‘ in code there will always be a right hand parenthesis ‘)’ following soon after. You can think of parentheses in code like hamburger buns- whenever there is one facing one way you’ll find the meat of the code between them and then another parenthesis on the other side of the meat. Here’s an example of parenthesis usage-
1 2 3 4 5 6 7 8 |
void loop() // often you'll find empty parenthesis, // if there was info to pass it would go between them { if( movement == true ) // these parenthesis are asking a question { sparki.moveForward( 30 ); // these parentheses are passing information } } |
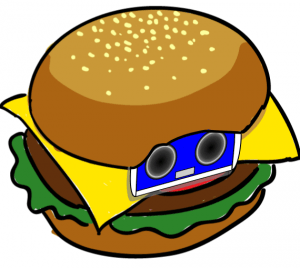
What? Robots in burgers are totally normal.
Curly Brackets: (Yummy!)
Curly brackets are used to let the robot or computer know when a chunk of code that belongs to a particular function or command begins and ends. This is especially useful with looping code since the robot or computer needs to know when to go back to the beginning of the looping section and start over. For loops are a perfect example of this usage of curly brackets. Curly brackets are also used for sections of non-looping code so that the computer knows how much code to skip over when that section of code will not be executed. If statements are a perfect example of this usage of curly brackets. You can also think of curly brackets in code like hamburger buns- whenever there is one facing one way you’ll find the meat of the code between them and then another curly bracket on the other side of the meat. You’ll also often see sets of curly brackets inside of curly brackets! This is called “nested” brackets- it’s kind of like a quadruple (or more) layer hamburger because there are always an even number of hamburger buns, never an odd number! Here’s an example of curly bracket usage and nested curly brackets:
1 2 3 4 5 6 7 8 9 10 11 |
void loop() { // this is the first curly bracket that tells the robot to // execute the code inside these curly brackets if( movement == true ) { // first nested bracket (inside of loop brackets) // if movement is not equal to true code inside // these brackets will be skipped sparki.moveForward( 30 ); } // closing nested curly backet (inside of loop brackets) } // this is the closing curly bracket that tell the robot it // is done with the loop section of code |
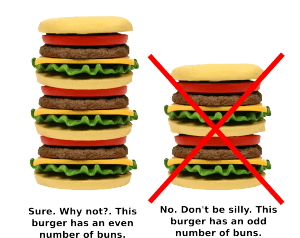