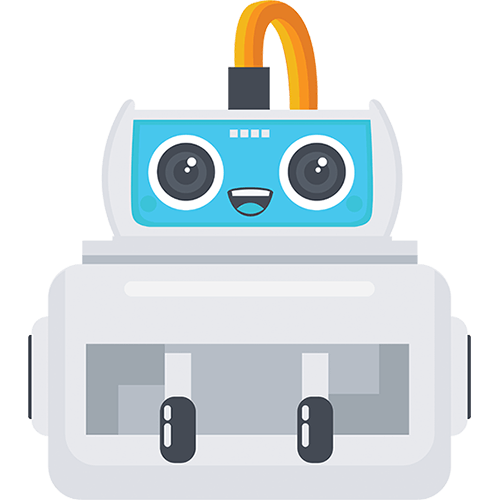
Writing code can be hard. This lessons is dedicated to more tips to help you write code with fewer error messages.
Here’s what we’ll be covering on this page:
With pseudocode you can concentrate on the problem you are trying to solve. There’s no need to declare
variables, use semicolons or even curly brackets. After figuring out the outline of what your code should look like with pseudocode you will go back and fill those in when you actually write the code.
The most important thing when you are writing pseudocode is to get all the main ideas that you need to do down on paper. This way you can figure out the order that things need to happen and it will be more obvious when you’re forgetting to check a sensor or turn a motor.
The circle is where the program or robot starts.
Squares are actions that the program or robot takes which don’t require making a decision or looping.
Diamonds are places where the program or robot needs to make a decision. If the answer to the question in the diamond is “yes” then the program or robot follows the arrow labeled “yes.” If the answer to the question in the diamond is “no” then the program or robot follows the arrow labeled “no.”
You should decide which of the two, pseudocode or Logic Flow Charts, you prefer. Of course, you can always use a mix of the two. (That’s probably the best way to go.) Get in the habit of using these tools. They are useful for planning and explaining how your code works (or how you want it to work) to other people.
Pseudocode
Before they sit down to write code most programmers write something called pseudocode. Pseudocode is a way to write code that doesn’t need to be correct (in fact it never is) but it allows the coder to make a map for how the code should work. Here’s an example of some pseudocode for an automated barbecue machine:
1 2 3 4 5 6 |
IF time is up OR the temp has reached 350°- turn off BBQ fire and beep ELSE execute the stuff below turn the meat in the BBQ drip sauce on the meat check the temperature of the meat ADD one to the timer |
Pseudocode Helps You Create the Meat of Your Code
There is no correct way to write pseudocode. I tend to write mine with the words that indicate a type of code like IF, ELSE and OR capitalized, but you don’t have to do that if you don’t want. As you practice writing pseudocode you’ll figure out what works best for you. You could have just as easily written the pseudocode above like this:
1 2 3 4 5 6 7 8 9 10 11 |
if (time is up OR the temp has reached 350°) { turn off BBQ fire and beep } else { turn the meat in the BBQ drip sauce on the meat check the temperature of the meat ADD one to the timer } |
Logic Flow Charts
Programmers will also write pseudocode using something called Logic Flow Charts. These are similar to pseudocode but they use circles, squares and diamonds with lines between them instead of text, like pseudocode. The lines between the pieces have arrows that indicate which way the code, or logic, is supposed to flow. Here’s and example of a Logic Flow Chart that does the same thing as the pseudocode above: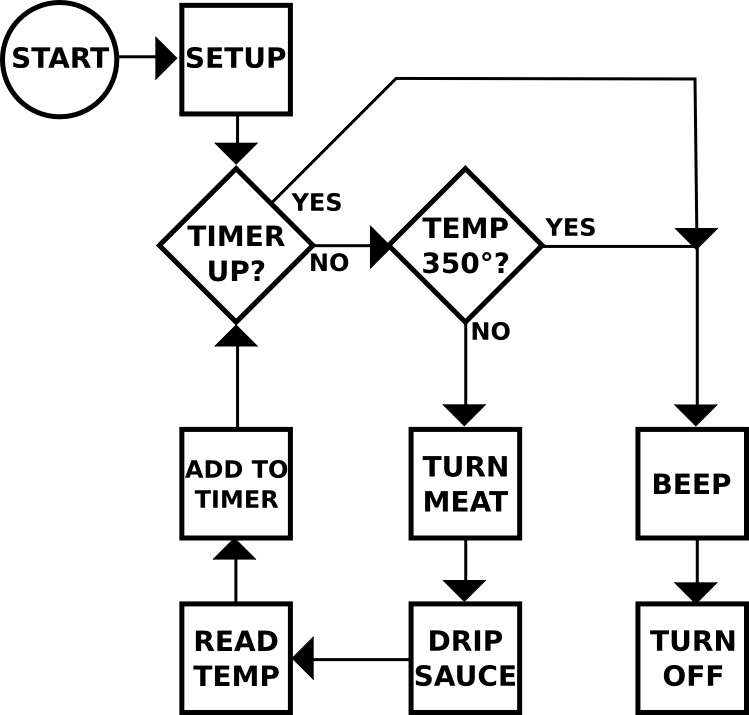
BBQ Making Robot Logic Flow Chart
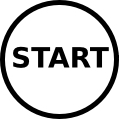
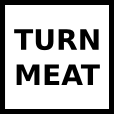
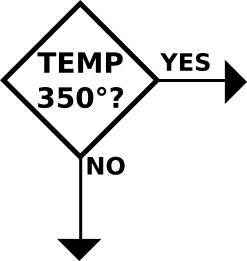
Writing Code Slowly
Another habit you should get used to is writing code slowly. It’s important to write just a small, simple bit of code first. Then you can check it to make sure it works the way you think it should. Then you can add another small piece of code and see how adding that to your code changes everything. This way when your code breaks you know exactly what made it break. This one is really hard to get used to and use. It’s very tempting to write a whole bunch of code and test it out. Often you’ll run into error messages or problems with the logic flow of your code when you do this. Sometimes trying to track down the problem will take up way more time that it would have taken if you had just written the code slowly, a piece at a time.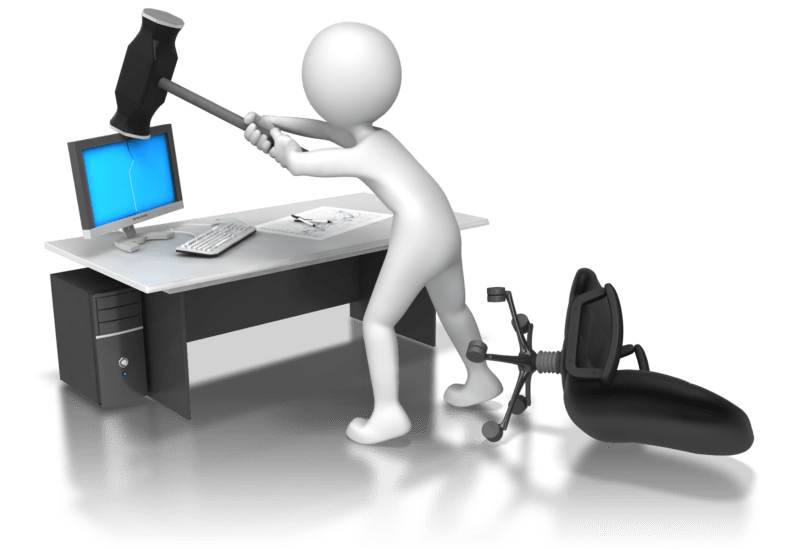
Coding Can Be Frustrating, Make It Easier on Yourself by Taking it Slowly
All coders are told to take a piece at a time when they start coding. We all say “yeah, yeah, yeah” but rarely take the advice. Usually coders just have to experience a couple really frustrating attempts at writing code to start using this advice. If you start using it now you’ll be ahead of all the people who said “yeah, yeah, yeah!”Using Example Code
Using example code can be really useful. It’s definitely ok to take example code and change it so it works with your robot or does what you want. A lot of people do that. There are a couple things you should know about example code before you start throwing it around, though. The first is that you will always learn more and become a better coder if you write the code yourself. Even if that just means copying some example code by typing it out again instead of copying and pasting. Writing the code yourself will take a lot of time, though. You need to be able to decide which is more valuable- getting the project done quickly or having a better understanding of the code running in your project. The second thing that you need to bear in mind is that anyone can write example code and post it on the internet. You never know if that person is a wiz at coding or if they are a newbie just figuring out how to use if statements. Make sure you do a little research to make sure that the place or person supplying the code knows what they are talking about.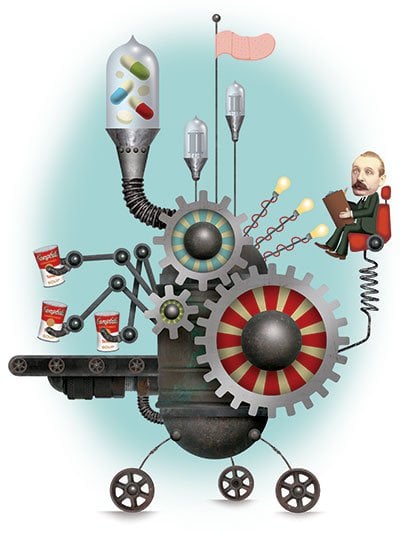
The InterGalacticCombobulatorX8.0
Last of all, make sure that you know what kind of hardware the example code was written to run. If the example code was written for an InterGalacticCombobulatorX8.1 and you have an InterGalacticCombobulatorX8.2 it may not work on the hardware you have! Even worse, some parts of the example code may work, but other parts may not work. This might make you think that all of the code should work and you can waste hours or even days scratching your head!Comment, Comment, Comment!
You may not think you need comments- trust me, you do. There are so many reasons to use comments that I can’t tell you this enough. If you ever want to share your code it needs to have a ton of useful comments in it so whoever picks up the code can figure it out. This is important if you want to work on a team to make a project or you ever need help. It’s lots of fun to work on projects with other people and really useful to have someone else look over your code, so comment, comment, comment! You also might put down your project for a little bit in order to work on something else. When you come back to your project it may be a week, a month or even a couple years later. If there are no comments you’ll have to remember what you were thinking when you wrote the code! That can take a little bit of time and may cause some confusion. The way around this issue? You guessed it. Comment, comment, comment!
1 |
// Use Comments all the time! |
Recap of What We’ve Covered so Far
orNext Step:
There are just a couple more things to cover in this basic coding introduction- the different ways that robot sensors and motors work.