Sparki has a small red LED light that can be used to display simple information. You can turn it on and off using digitalWrite, or partially turn it on using analogWrite.
You can also use the analogWrite function to tun the LED partially on or off:
SparkiDuino already has code examples for you to use: File > Examples > Status_LED
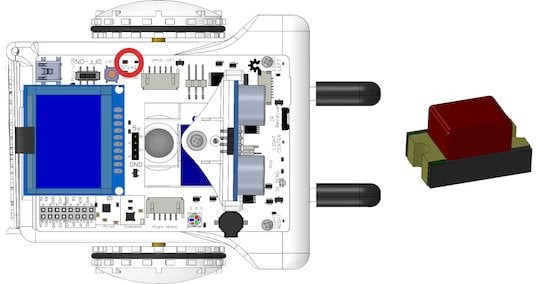
Using the Part
With the basic Sparki code in place, you can use the status LED using these commands. You can copy-paste them into the code:
1 2 |
digitalWrite(STATUS_LED, HIGH); // will turn the LED on digitalWrite(STATUS_LED, LOW); // will turn the LED off |
1 2 3 |
analogWrite(STATUS_LED, 0); // will turn the LED 0% on (off) analogWrite(STATUS_LED, 123); // will turn the LED 50% on analogWrite(STATUS_LED, 255); // will turn the LED 100% on |
SparkiDuino already has code examples for you to use: File > Examples > Status_LED
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/******************************************* Status LED test Sparki has a red status LED, next to the power on LED. This code first blinks it on then off, then fades it on then off. ********************************************/ #include <Sparki.h> // include the sparki library void setup() { } void loop() { digitalWrite(STATUS_LED, HIGH); // will turn the LED on delay(500); // wait 0.5 seconds digitalWrite(STATUS_LED, LOW); // will turn the LED off delay(500); // wait 0.5 seconds for(int i=0; i<255; i++) // pulse the LED on { analogWrite(STATUS_LED, i); delay(5); } for(int i=255; i>0; i--) // pulse the LED off { analogWrite(STATUS_LED, i); // will turn the LED 100% on delay(5); } } |