Little Containers for Information
Here’s what we’ll be going over in this lesson about variables:Creating Variables
Computers work with information. But they really only work with two pieces of information- a one and a zero. In order to work with larger pieces of information the computer uses things called “variables” which are made up of a lot of those little ones and zeros. They are called variables because the information inside of them varies. You can think of variables as containers for information. The information (also called data) that goes inside the variable is called many different things- it’s usually referred to as a “value” or “data”. You can think of the variable as a container, like a mailbox, and the data that goes inside the variables are like mail that gets placed inside a mailbox. (We’ll use many different container examples through out this variable lessons.)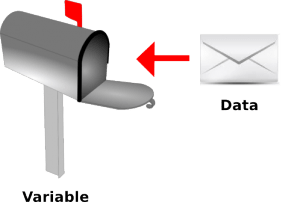
(Usually) You can change the value of the data inside the variable, use a variable in a mathematical equation (just like algebra, because it is algebra) and control all sorts of things using variables. Let’s look at the command sparki.moveForward, which makes Sparki move forward, as an example.
1 |
sparki.moveForward(); |
1 |
sparki.moveForward(10); |
1 2 |
int distance = 10; sparki.moveForward(distance); |
1 |
int distance; // an empty variable named distance for use later |
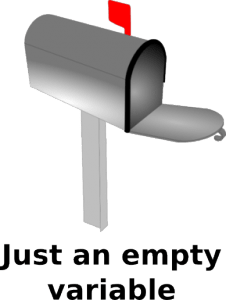
(One note- be careful not to name your variable any “reserved” word. That’s a word that’s already used for something in the programming language. For example, you would never want to name your variable something like “int” since int is already used to create an integer. If you accidentally use a reserved word you’ll get an error and you can simply change the variable name. Reserved words are often highlighted in a different color to help you understand that they are already in use. Handy, right?) Only after you’ve declared a variable can you put data into it. Although, you can declare a variable and assign a value in the same line of code as you saw above.
Changing Variables: Also Called “Reassigning”
You may be asking, why don’t people just use the number ten then, instead of a variable? After all, “10” is only two characters and “distance” is eight- also you had to write that whole extra line of code to create (also called “declaring” a variable) the variable. Let’s look at some ways that variables can come in handy.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include <Sparki.h> // include the sparki library int distance = 10; void setup() // code inside these brackets runs first, and only once { //empty setup } void loop() { sparki.moveForward(distance); delay(1000); //wait one second distance = 5; } |
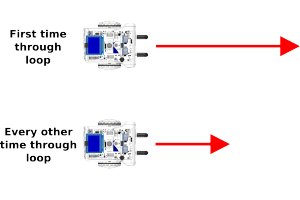
In the code above the first time through the loop Sparki will move forward a distance of ten centimeters. Then the “delay” command tells Sparki to hang out doing nothing for 1000 milliseconds (or one regular second). After that the data in the variable “distance” is changed (or “reassigned”) to a value of five. Now, when Sparki goes through the loop of code a second time Sparki will only move forward five centimeters. You don’t have to use the word “int” again since the computer already understands that the variable is an integer. So, by manipulating the variable you can make changes in the robot’s actions. (There’s a lot of other stuff like setup and loop, if you don’t understand those click here.) Let’s take it a little further:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include <Sparki.h> // include the sparki library int distance = 10; void setup() // code inside these brackets runs first, and only once { //empty setup } void loop() { sparki.moveForward(distance); delay(1000); //wait one second distance = distance + 5; //add five to whatever value distance is currently } |
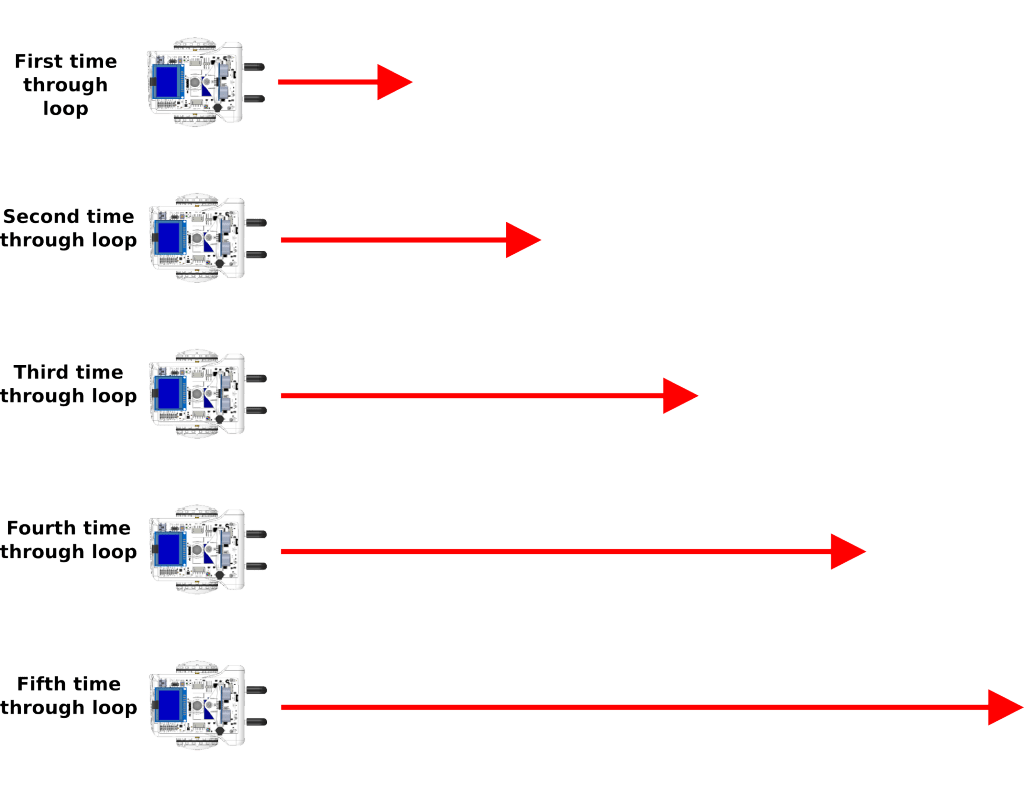
Now you may be starting to see why variables are so powerful. In the code above Sparki will add five centimeters to the distance it travelled forward last time. Without a variable to change the amount of code we would have to write to recreate this simple program would be enormous! Here’s a start on writing this program (just the loop portion) without using a variable to give you an idea of what I mean:
1 2 3 4 5 6 7 8 9 10 11 |
void loop() { sparki.moveForward(10); delay(1000); //wait one second sparki.moveForward(15); delay(1000); sparki.moveForward(20); delay(1000); sparki.moveForward(25); delay(1000); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include <Sparki.h> // include the sparki library int distance = 10; void setup() // code inside these brackets runs first, and only once { //empty setup } void loop() { sparki.moveForward(distance); delay(distance * 100); //wait one second (the first time through) distance = distance + 5; //add five to whatever value distance is currently } |
1 |
distance = distance + 5; //add five to whatever value distance is currently |
1 |
distance += 5; //add five to whatever value distance is currently |
1 2 3 4 5 |
distance = distance + 1; //we could write this distance ++; //but why would we when we could write this? distance = distance = 1; //it works for subtraction too distance --; |
Recap of What We’ve Covered So Far
or keep going and learn about:Different Kinds of Variables
Variable types
In the C language, variables can only store one type of data. If you need to store multiple types of data, then you need multiple types of variables: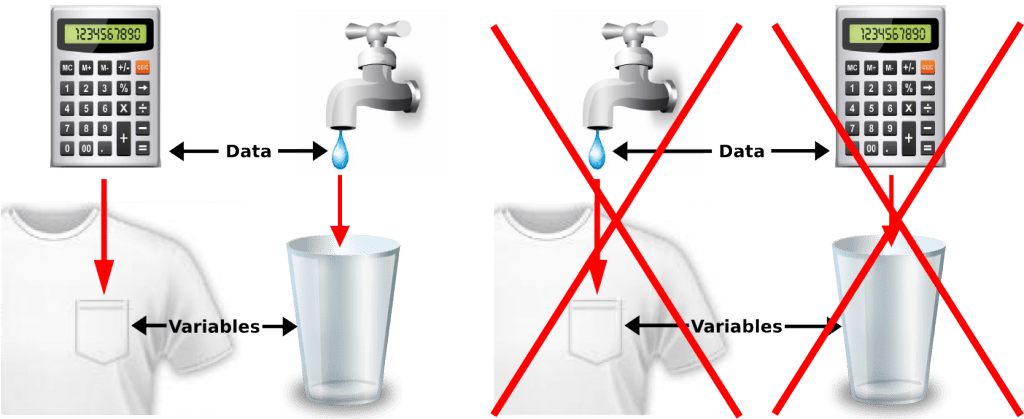
Just like there are different types of containers for different things in real life there are different variables types (or containers) in programming. You would never use your pocket to hold water and you would never use a water balloon to keep your calculator in, would you? That wouldn’t make sense. Just like the example above we use “int” type variables to hold integers. A variable type called “char” is used to hold a single character such as a letter or a punctuation mark (it can even hold weird ones like µ, € or °) and the variable type “float” is used to hold a number with a decimal point. You will use the words “int,” “char” and “float” to declare variables of each of these types. Here’s some more information about each of these types of variables: Booleans- bool Integer Numbers – int Floating Point Numbers – float Characters – char A couple more thing to note is that can put an integer value into a float variable. You also can put a float value into an integer variable, the computer just throws away the decimal point and anything that comes after it. Think of it like this- you can store two different materials water and sugar in a glass and you can also store them both in a water balloon. (Just not at the same time if you expect to get them back out in the original form.)
Variable Size
Each variable type has a different size. This means that you can’t put an enormous number into an integer variable because it won’t fit, there’s not enough space to store the value. Going back to our mailbox example, it’s kind of like trying to stuff a really big envelope containing something more than just mail (maybe a robot or an inflatable pool) into a mailbox. It just won’t fit. Here are the values that the three variables we are learning about can contain in SparkiDuino:- Integer (int) – any number between -32,768 to +32,767
- Float (float) – can be as large as 3.4028235E+38 and as small as -3.4028235E+38. Float values can also only have 6 to 7 digits of precision, meaning that there can only be 6 or 7 numbers after the decimal point (Scientific notation as shown above allows for an exception to this rule.)
- Character (char) – can hold any single character you can create with your keyboard
Arrays
And finally, to help store and manage multiple values of a variable we’ll be talking about Arrays – [] Arrays are similar to a bunch of school lockers in a row. Each of the individual lockers in the row can contain a single backpack or variable value. (I know backpacks and locker size differ, for the sake of argument let’s say they’re all the same size in our array example.) Also- each array can only contain one type of data. You can’t store water in the row of school lockers no matter how hard you try, No putting character values into a bunch of integer school lockers! That’s like putting a hose into the lockers and expecting to be able to come back later to get a quick drink from the water you tried to store in the lockers. It won’t work.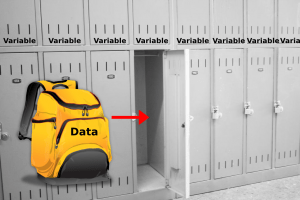