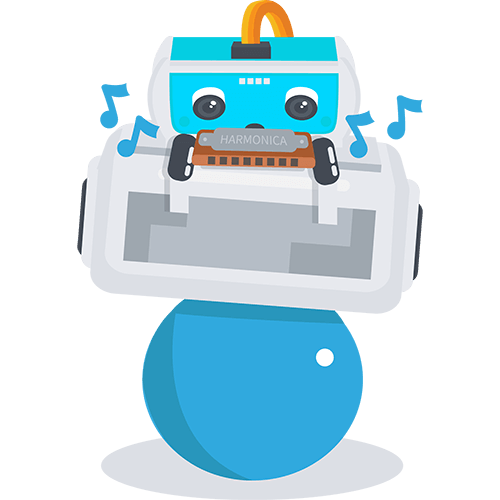
Making Music with the Accelerometer
Introduction
Although we have used different sensors like the ultrasonic distance ranger, the light sensors, or the line following sensors, there are more Sparki sensors to discover. One of them is the 3D accelerometer. The accelerometer can be used for a lot of things, but in this lesson, we will start to learn about it with a simple goal in mind: make music by moving Sparki with our hands.What You’ll Need
- A Sparki.
How It Works
If you are not familiar with Sparki’s accelerometer, please first read this description. It is one of Sparki’s more complex parts. The accelerometer commands in SparkiDuino give the acceleration acting on Spark in X, Y and Z axes. Here is a map of those Axes: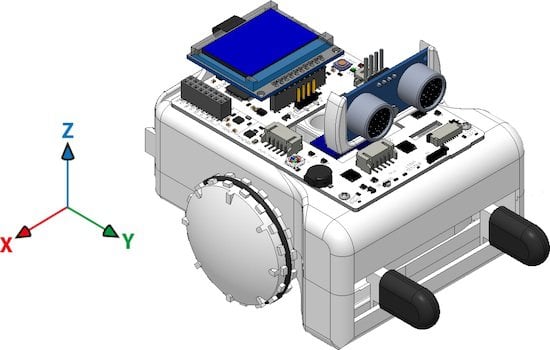
1 2 3 |
sparki.accelX(); sparki.accelY(); sparki.accelZ(); |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/******************************************* Basic Accelerometer Sensor test Sparki has a 3-Axis accelerometer. It is used to detect the acceleration Sparki is experiencing. Usually, this mostly means the force of gravity. It can do this in all 3 XYZ axis, which are left/right (X), forward/backwards (Y), and up/down (Z). This is the same part that smartphones use to tell how you’re tilting them. This program shows how to read the sensor and display the information on the LCD. ********************************************/ #include <Sparki.h> // include the sparki library void setup() { } void loop() { sparki.clearLCD(); // wipe the screen float x = sparki.accelX(); // measure the accelerometer x-axis float y = sparki.accelY(); // measure the accelerometer y-axis float z = sparki.accelZ(); // measure the accelerometer z-axis // write the measurements to the screen sparki.print("Accel X: "); sparki.println(x); sparki.print("Accel Y: "); sparki.println(y); sparki.print("Accel Z: "); sparki.println(z); sparki.updateLCD(); // display all of the information written to the screen delay(100); } |
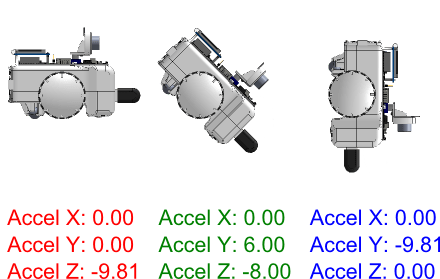
- Read the Y acceleration value from the accelerometer.
- Make that value to always be positive (since frequencies can not be negative!) using the abs function.
- Multiply that value for a number. The number should be big enough to convert our Y reading in a frequency corresponding to an audible sound that can be sent to the buzzer. For example: a frequency of 440 Hz is the A note. So, let’s start multiplying the reading by 100. Thus if Sparki is fully vertical (in the Y axis), the frequency will be near 1000 Hz (or 1 KHz), since 9.8 x 100 = 980.
- Wait for some milliseconds (note’s duration) using the delay function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include <Sparki.h> // include the sparki library void setup() { } void loop() { sparki.clearLCD(); // wipe the screen float x = sparki.accelX(); // measure the accelerometer x-axis float y = sparki.accelY(); // measure the accelerometer y-axis float z = sparki.accelZ(); // measure the accelerometer z-axis // write the measurements to the screen sparki.print("Accel X: "); sparki.println(x); sparki.print("Accel Y: "); sparki.println(y); sparki.print("Accel Z: "); sparki.println(z); sparki.updateLCD(); // display all of the information written to the screen sparki.beep(abs(y)*100); delay(300); // note duration } |
1 |
sparki.beep(abs(y)*100); |
1 |
delay(abs(x)*50 + 100); // note duration: the 100 number is there to prevent zero duration notes. |
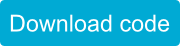