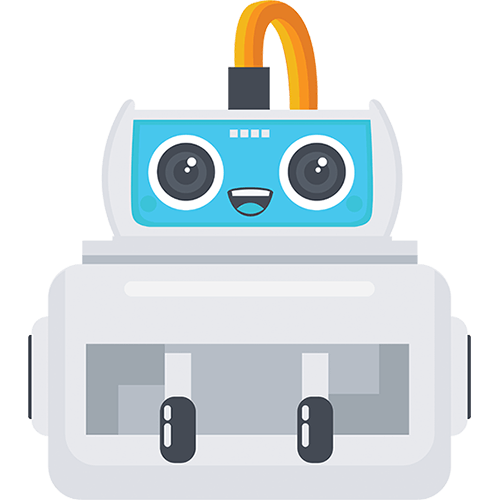
Floating point numbers are numbers that have decimal points. Unlike integers, floating point numbers let you use partial numbers. This means they are good to use when you do not know if there will be numbers after a decimal point, like when you divide and keep the remainder. Since “floating point numbers” is a mouthful to use every time programmers want to refer to these types of numbers this type of variable is usually called a “float.”
Floats can also can be much bigger than integers. As big as 2^128 or small as 2^-127. That’s very, very big, for example:
340,282,340,000,000,000,000,000,000,000,000,000,000.0
or very, very small:
0.000000000000000000000000000000000000000000000014012985
Here’s what we’ll be covering on this page:
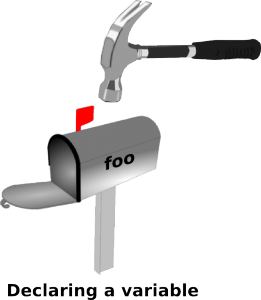
This will create a float named “foo” with a stored value of 0.0.
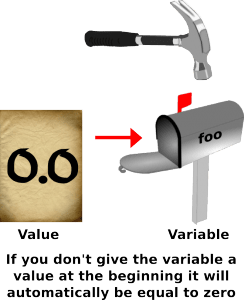
You can think of a float variable like a mailbox with a letter in it. You can write any number on the paper inside the letter and the robot will look inside the letter anytime it sees the variable name that is on the mailbox. Then the robot will just insert whatever number is on the letter into the code it is running in place of the variable.
You can also declare a float and assign a number to the variable in the same line of code. Here’s what it looks like to declare a float variable named “foo ” with the initial value of six point seven three:
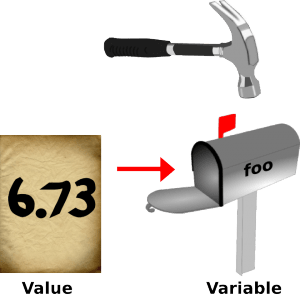
Before, when we divided 10 by 4 using an integer we got an answer of 2. You will see that foo/blerg now gives the correct result since we used a float!
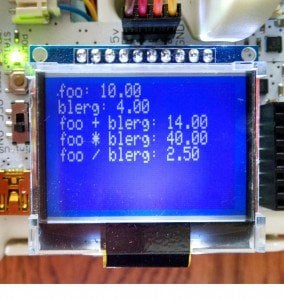
Declaring a Float Variable
You create a float variable by declaring it in the same way that you declare an integer, except instead of using int you use float:
1 |
float foo; // declaring a float variable named foo |
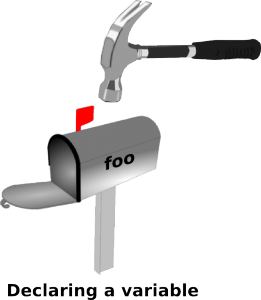
This will create a float named “foo” with a stored value of 0.0.
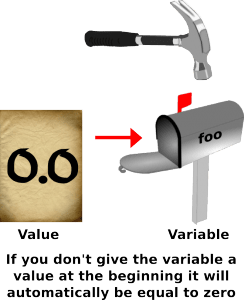
Assigning Values (Putting Information in the Float Variables)
You can then change the float value by assigning a value to the variable. (Just like you did with integers.) This is done simple by writing the variable name followed by an equals sign, followed by the value you want to put in the variable. Then you end your command for assigning a variable with the all important semicolon. Everything else is pretty self explanatory but the semicolon is there so the computer knows that it has reached the end of a line of instructions that should now be executed. You can think of the semicolon as a green light that tells the computer it has all the parts of a command and it can start moving ones and zeros around. Here’s what it looks like when we assign the value of eight point zero zero six to the float variable “foo.”
1 |
foo = 8.006; |
1 |
float foo = 6.73; // declaring a float named foo with a value of six point seven three |
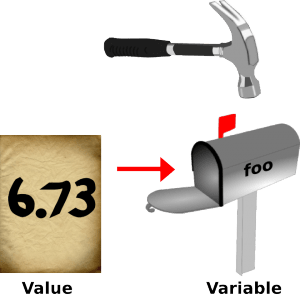
Doing Math with Floats
Now that you know about floats, try using them instead of integers with the code we covered before:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
#include <Sparki.h> // include the sparki library void setup() { } void loop() { sparki.clearLCD(); // erase the LCD float foo; // declares a variable of type integer with the name 'foo' foo = 10; // assigns the value of 10.0 to foo float blerg = 4; // declare a float with the value of 4.0 sparki.print( "foo: "); sparki.println( foo ); sparki.print( "blerg: "); sparki.println( blerg ); sparki.print( "foo + blerg: "); sparki.println( foo + blerg ); // foo added to blerg sparki.print( "foo * blerg: "); sparki.println( foo * blerg ); // foo multiplied times blerg sparki.print( "foo / blerg: "); sparki.println( foo / blerg ); // foo divided by blerg sparki.updateLCD(); // put what has been drawn onto the screen delay(1000); // wait 1 seconds (1000 milliseconds) } |
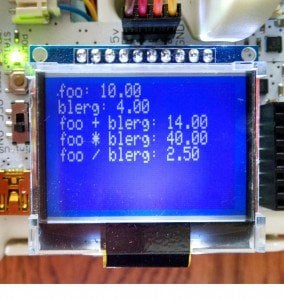